Php can connect to the mysql db in two ways. They are Using the mysqli extension and using PDO.
MYSQL db have two types such as object oriented mysqli and procedural mysqli
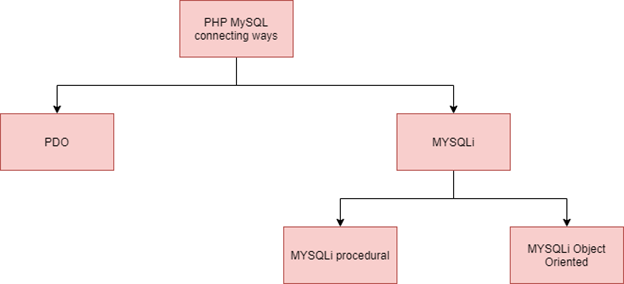
Difference between the MYSQli and PDO.
MYSQLi | PDO |
Only can work with the mysql database. | Can work with the towel difference data bases. |
Support prepared statement and it will protect the sql injection. | Support prepared statement and it will protect the sql injection. |
Process not much easy when switch the database | PDO make the process easy than mysli |
Object Oriented | Object Oriented |
Offered procedural API | Not offered procedural API |
Installation steps Link: http://php.net/manual/en/mysqli.installation.php | Installation steps Link: http://php.net/manual/en/pdo.installation.php |
Server connecting code for MYSQLi Object Oriented.
<?php
$servername = "localhost";
$username = "username";
$password = "password";
// Create connection
$conn = new mysqli($servername, $username, $password);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
echo "Connected Successfully";
?>
Close connection MYSQLi Object Oriented
$conn->close();
Server connecting code for MYSQLi Procedural.
Server connecting code for MYSQLi Object Oriented.
<?php
$servername = "localhost";
$username = "username";
$password = "password";
// Create connection
$conn = new mysqli($servername, $username, $password);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
echo "Connected Successfully";
?>
Server connecting code for MYSQLi Procedural.
<?php
$servername = "localhost";
$username = "username";
$password = "password";
// Create connection
$conn = mysqli_connect($servername, $username, $password);
// Check connection
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
echo "Connected Successfully";
?>
Close connection MYSQLi Procedural. mysqli_close($conn);
Server connecting code for PDO (PHP Data Objects).
<?php
$servername = "localhost";
$username = "username";
$password = "password";
try {
$conn = new PDO("mysql:host=$servername;dbname=myDB", $username, $password);
// set the PDO error mode to exception
$conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
echo "Connected Successfully";
} catch(PDOException $e) {
echo "Connection failed: " . $e->getMessage();
}
?>
Close connection PDO (PHP Data Objects). $conn = null;
The connection should close using above codes because when end the script connection should be close automatically.
For process this code the PHP version should be prior to 5.2.9 and 5.3. If not use this code for MYSQLi Object Oriented as a connector.
if (mysqli_connect_error()) {
die("Database connection failed: " . mysqli_connect_error());
}
PHP and Mongo DB connection.
- Install and configure the mongo DB using this link.
- Now create the database.
use newdb
- Now add dummy details to the db.
- Now connect the db using php code bellow.
?php
$mng = new MongoDB\Driver\Manager("mongodb://localhost:27017");
$qry = new MongoDB\Driver\Query([]);
$rows = $mng->executeQuery("newdb.products", $qry);
foreach ($rows as $row) {
foreach ($rows as $row) {
echo nl2br("$row->item : $row->qty\n");
}
}
?>
How to downlead the Oracle database.
- We can download oracle database by using this link : Download Link.
- After installing the software create the connection using php as below code.
- In here connect function is using to connect the code to php and close function is using to close the connection.
$conn = oci_connect("phphol", "welcome", "//localhost/orcl");
if (!$conn) {
$m = oci_error();
echo $m['message'], "\n";
exit;
}
else {
print "Connected to Oracle!";
}
// Close the Oracle connection
oci_close($conn);
After these process using below url, we can display the output in the browser.
http://localhost/~phphol/connect.php
One thought on “MYSQL db and Mongo db How to connect PHP code to.”